Tiles API
List the tiles
Lists the tilesets from the tiles directory. Can be paginated with offset and limit parameters, otherwise first 50 items are returned.
Request
Query Parameters
Parameters | Type | Description |
---|---|---|
offset | integer |
The starting position of returned list of items.
Example: 10 Default: 0 Added in v4.2.0 |
limit | integer |
Maximum number of items which will be returned.
Example: 50 Default: 50 Added in v4.2.0 |
Responses
Code | Content | Description |
---|---|---|
200 | application/json | TileList |
Rescan the tiles
Rescans tiles directory. Does NOT remove dead files.
Request
Responses
Code | Content | Description |
---|---|---|
200 | application/json | TileList |
Reload the tiles
Rescans the tiles directory and automatically removes dead files. Optionally may remove remote tile records.
Request
Query Parameters
Parameters | Type | Description |
---|---|---|
removeRemote | boolean |
Removes remote tile records. Does not remove files from the remote server.
Example: true Default: false Added in v4.6.0 |
Responses
Code | Content | Description |
---|---|---|
200 | application/json | TileRemove |
Add remote tile
Adds MTPKG remote tile record.
Request
Body
Content-Type | Data | application/json |
---|
Responses
Code | Content | Description |
---|---|---|
200 | application/json | TileItem |
400 | application/json | Errors |
404 | application/json | Errors |
Detail of the tileset
Gets information about the given tileset.
Request
Path Parameters
Parameters | Type | Description |
---|---|---|
id | string |
Identifier of the tileset
Example: b142543e-ffa2-4994-acbc-825012e75a97 Added in v4.2.0 |
Responses
Code | Content | Description |
---|---|---|
200 | application/json | TileItemDetail |
400 | application/json | Errors |
404 | application/json | Errors |
Change metadata of the given tileset
Changes metadata (name, published) of the given tileset.
Request
Path Parameters
Parameters | Type | Description |
---|---|---|
id | string |
Identifier of the tileset
Example: b142543e-ffa2-4994-acbc-825012e75a97 Added in v4.2.0 |
Body
Content-Type | Data | application/json |
---|
Responses
Code | Content | Description |
---|---|---|
200 | application/json | TileItemDetail |
400 | application/json | Errors |
404 | application/json | Errors |
Delete the tileset
Deletes the given tileset. Before deleting, it is automatically unpublished and cannot be served anymore.
Request
Path Parameters
Parameters | Type | Description |
---|---|---|
id | string |
Identifier of the tileset
Example: b142543e-ffa2-4994-acbc-825012e75a97 Added in v4.2.0 |
Responses
Code | Content | Description |
---|---|---|
200 | application/json | TileRemove |
400 | application/json | Errors |
404 | application/json | Errors |
Publish the tileset
Publishes the given tileset. Does nothing, if the tileset is already published.
Request
Path Parameters
Parameters | Type | Description |
---|---|---|
id | string |
Identifier of the tileset
Example: b142543e-ffa2-4994-acbc-825012e75a97 Added in v4.2.0 |
Responses
Code | Content | Description |
---|---|---|
200 | application/json | TileItemDetail |
400 | application/json | Errors |
404 | application/json | Errors |
Unpublish the tileset
Unpublishes the given tileset. Does nothing, if the tileset is already unpublished.
Request
Path Parameters
Parameters | Type | Description |
---|---|---|
id | string |
Identifier of the tileset
Example: b142543e-ffa2-4994-acbc-825012e75a97 Added in v4.2.0 |
Responses
Code | Content | Description |
---|---|---|
200 | application/json | TileItemDetail |
400 | application/json | Errors |
404 | application/json | Errors |
TileList
Property | Type | Description |
---|---|---|
items | array[TileItem ] |
TileItem
Tile item
Property | Type | Description |
---|---|---|
id | string | Identifier of the tileset
Example: b142543e-ffa2-4994-acbc-825012e75a97 |
name | string | Name of the tileset
Example: maptiler-osm |
title | string | Title of the tileset
Example: Maptiler OSM Data |
fileName | string | File name
Example: maptiler-osm.mbtiles |
published | boolean | Says whether the tileset is published
Example: true |
type | string | Type of the data
Example: mbtiles Allowed values:
geopackage
mbtiles
mtpkg
postgis
virtual |
tileType | string | File type of the data
Example: vector Allowed values:
raster
terrain
vector |
TileItem example
{
"id": "b142543e-ffa2-4994-acbc-825012e75a97",
"name": "maptiler-osm",
"title": "Maptiler OSM Data",
"fileName": "maptiler-osm.mbtiles",
"published": true,
"type": "mbtiles",
"tileType": "vector"
}
TileRemove
Property | Type | Description |
---|---|---|
removed | array [string] | The names of removed tilesets
|
TileRemove example
{"removed": ["maptiler-osm"]}
TileAdd
Property | Type | Description |
---|---|---|
storage | string | Storage type of remote server
Example: http Allowed values:
http
s3 |
url | string | URL of remote file
Example: https://data.maptiler.com/downloads/tileset/europe/switzerland/zurich.mtpkg |
TileAdd example
{
"storage": "http",
"url": "https://data.maptiler.com/downloads/tileset/europe/switzerland/zurich.mtpkg"
}
Errors
Property | Type | Description |
---|---|---|
errors | Error |
Error
Error message
Property | Type | Description |
---|---|---|
message | string | Example: Error message |
Error example
{"message": "Error message"}
TileItemDetail
Tile details
Property | Type | Description |
---|---|---|
id | string | Identifier of the tileset
Example: b142543e-ffa2-4994-acbc-825012e75a97 |
name | string | Name of the tileset
Example: maptiler-osm |
title | string | Title of the tileset
Example: Maptiler OSM Data |
fileName | string | File name
Example: maptiler-osm.mbtiles |
published | boolean | Says whether the tileset is published
Example: true |
type | string | Type of the data
Example: mbtiles Allowed values:
geopackage
mbtiles
mtpkg
postgis
virtual |
tileType | string | File type of the data
Example: vector Allowed values:
raster
terrain
vector |
urls | TileItemDetailUrls |
TileItemDetail example
{
"id": "b142543e-ffa2-4994-acbc-825012e75a97",
"name": "maptiler-osm",
"title": "Maptiler OSM Data",
"fileName": "maptiler-osm.mbtiles",
"published": true,
"type": "mbtiles",
"tileType": "vector",
"urls": {
"embeddable": "http://localhost:3650/api/tiles/maptiler-osm",
"ogcTiles": "http://localhost:3650/api/tiles/maptiler-osm/tiles",
"tilejson": "http://localhost:3650/api/tiles/maptiler-osm/tiles.json",
"wmts": "http://localhost:3650/api/tiles/maptiler-osm/WMTSCapabilities.xml",
"xyz": "http://localhost:3650/api/tiles/maptiler-osm/{z}/{x}/{y}"
}
}
TileItemDetailUrls
Accessible URLs for the tileset
Property | Type | Description |
---|---|---|
embeddable | string | URL for embeddable viewer
Example: http://localhost:3650/api/tiles/maptiler-osm |
ogcTiles | string | URL for OGC API - Tiles
Example: http://localhost:3650/api/tiles/maptiler-osm/tiles |
tilejson | string | URL for tileJSON / layerJSON
Example: http://localhost:3650/api/tiles/maptiler-osm/tiles.json |
wmts | string | URL for WMTS
Example: http://localhost:3650/api/tiles/maptiler-osm/WMTSCapabilities.xml |
xyz | string | URL for XYZ
Example: http://localhost:3650/api/tiles/maptiler-osm/{z}/{x}/{y} |
TileItemDetailUrls example
{
"embeddable": "http://localhost:3650/api/tiles/maptiler-osm",
"ogcTiles": "http://localhost:3650/api/tiles/maptiler-osm/tiles",
"tilejson": "http://localhost:3650/api/tiles/maptiler-osm/tiles.json",
"wmts": "http://localhost:3650/api/tiles/maptiler-osm/WMTSCapabilities.xml",
"xyz": "http://localhost:3650/api/tiles/maptiler-osm/{z}/{x}/{y}"
}
TileChange
Property | Type | Description |
---|---|---|
name | string | New name
Example: maptiler-osm |
published | boolean | New state of the tile serving
Example: true |
TileChange example
{"name": "maptiler-osm", "published": true}
TileItemDetailUrls
Accessible URLs for the tileset
Property | Type | Description |
---|---|---|
embeddable | string | URL for embeddable viewer
Example: http://localhost:3650/api/tiles/maptiler-osm |
ogcTiles | string | URL for OGC API - Tiles
Example: http://localhost:3650/api/tiles/maptiler-osm/tiles |
tilejson | string | URL for tileJSON / layerJSON
Example: http://localhost:3650/api/tiles/maptiler-osm/tiles.json |
wmts | string | URL for WMTS
Example: http://localhost:3650/api/tiles/maptiler-osm/WMTSCapabilities.xml |
xyz | string | URL for XYZ
Example: http://localhost:3650/api/tiles/maptiler-osm/{z}/{x}/{y} |
TileItemDetailUrls example
{
"embeddable": "http://localhost:3650/api/tiles/maptiler-osm",
"ogcTiles": "http://localhost:3650/api/tiles/maptiler-osm/tiles",
"tilejson": "http://localhost:3650/api/tiles/maptiler-osm/tiles.json",
"wmts": "http://localhost:3650/api/tiles/maptiler-osm/WMTSCapabilities.xml",
"xyz": "http://localhost:3650/api/tiles/maptiler-osm/{z}/{x}/{y}"
}
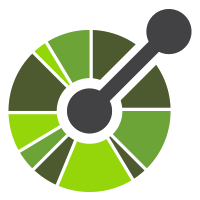