Maps API
List maps
Added in v4.3.0
GET http://localhost:3650/api/v1/admin/maps
Lists maps from maps directory. It can be paginated with offset and limit parameters, otherwise first 50 items are returned.
Request
Query Parameters
Parameters | Type | Description |
---|---|---|
offset | integer |
The starting position of returned list of items.
Example: 10 Default: 0 Added in v4.3.0 |
limit | integer |
Maximum number of items which will be returned.
Example: 50 Default: 50 Added in v4.3.0 |
Responses
Code | Content | Description |
---|---|---|
200 | application/json | MapList Object |
400 | application/json | Errors Object |
Detail of the map
Added in v4.3.0
GET http://localhost:3650/api/v1/admin/maps/{id}
Gets information about the given map.
Request
Path Parameters
Parameters | Type | Description |
---|---|---|
id | string |
Identifier of the map.
Example: streets Added in v4.3.0 |
Responses
Code | Content | Description |
---|---|---|
200 | application/json | MapItemDetail Object |
400 | application/json | Errors Object |
404 | application/json | Errors Object |
Change metadata of the map
Added in v4.3.0
POST http://localhost:3650/api/v1/admin/maps/{id}
Changes metadata (format, quality, published) of the given map.
Request
Path Parameters
Parameters | Type | Description |
---|---|---|
id | string |
Identifier of the map.
Example: streets Added in v4.3.0 |
Body
Content-Type | Data | application/json |
---|
Responses
Code | Content | Description |
---|---|---|
200 | application/json | MapItemDetail Object |
400 | application/json | Errors Object |
404 | application/json | Errors Object |
Delete the map
Added in v4.3.0
DELETE http://localhost:3650/api/v1/admin/maps/{id}
Deletes the given map. Before deleting, it is automatically unpublished and cannot be served anymore.
Request
Path Parameters
Parameters | Type | Description |
---|---|---|
id | string |
Identifier of the map.
Example: streets Added in v4.3.0 |
Responses
Code | Content | Description |
---|---|---|
200 | application/json | MapRemove Object |
400 | application/json | Errors Object |
404 | application/json | Errors Object |
Publish the map
Added in v4.3.0
POST http://localhost:3650/api/v1/admin/maps/{id}/publish
Publishes the given map. Does nothing, if the map is already published.
Request
Path Parameters
Parameters | Type | Description |
---|---|---|
id | string |
Identifier of the map.
Example: streets Added in v4.3.0 |
Responses
Code | Content | Description |
---|---|---|
200 | application/json | MapItemDetail Object |
400 | application/json | Errors Object |
404 | application/json | Errors Object |
Unpublish the map
Added in v4.3.0
POST http://localhost:3650/api/v1/admin/maps/{id}/unpublish
Unpublishes the given map. Does nothing, if the map is already unpublished.
Request
Path Parameters
Parameters | Type | Description |
---|---|---|
id | string |
Identifier of the map.
Example: streets Added in v4.3.0 |
Responses
Code | Content | Description |
---|---|---|
200 | application/json | MapItemDetail Object |
400 | application/json | Errors Object |
404 | application/json | Errors Object |
MapList Object
Property | Type | Description |
---|---|---|
items | array [ MapItem Object] |
MapItem Object
Map item
Property | Type | Description |
---|---|---|
id | string | Identifier of the map
Example: streets |
name | string | Name of the map
Example: streets |
title | string | Title of the map
Example: Streets |
published | boolean | Says whether the map is published
Example: true |
format | string | Selected format of the map rasterization
Example: jpeg Allowed values:
jpeg
png
webp |
example
{
"id": "streets",
"name": "streets",
"title": "Streets",
"published": true,
"format": "jpeg"
}
Errors Object
Property | Type | Description |
---|---|---|
errors | Error |
Error Object
Error message
Property | Type | Description |
---|---|---|
message | string | Example: Error message |
example
{"message": "Error message"}
MapItemDetail Object
Map detail
Property | Type | Description |
---|---|---|
id | string | Identifier of the map
Example: streets |
name | string | Name of the map
Example: streets |
title | string | Title of the map
Example: Streets |
published | boolean | Says whether the map is published
Example: true |
format | string | Selected format of the map rasterization
Example: jpeg Allowed values:
jpeg
png
webp |
quality | integer | Format quality of the map rasterization
Example: 92 |
urls | MapItemDetailUrls Object |
example
{
"id": "streets",
"name": "streets",
"title": "Streets",
"published": true,
"format": "jpeg",
"quality": 92,
"urls": {
"embeddable": "http://localhost:3650/api/maps/streets",
"ogcTiles": "http://localhost:3650/api/maps/streets/tiles",
"stylejson": "http://localhost:3650/api/maps/streets/style.json",
"tilejson": "http://localhost:3650/api/maps/streets/tiles.json",
"tilejson256": "http://localhost:3650/api/maps/streets/256/tiles.json",
"wms": "http://localhost:3650/api/maps/streets/wms?service=WMS&request=GetCapabilities",
"wmts": "http://localhost:3650/api/maps/streets/WMTSCapabilities.xml",
"xyz": "http://localhost:3650/api/maps/streets/{z}/{x}/{y}.jpg",
"xyz256": "http://localhost:3650/api/maps/streets/256/{z}/{x}/{y}.jpg"
}
}
MapItemDetailUrls Object
Accessible URLs for the map
Property | Type | Description |
---|---|---|
embeddable | string | URL for the embeddable viewer
Example: http://localhost:3650/api/maps/streets |
ogcTiles | string | URL for OGC API - Tiles
Example: http://localhost:3650/api/maps/streets/tiles |
stylejson | string | URL for styleJSON
Example: http://localhost:3650/api/maps/streets/style.json |
tilejson | string | URL for tileJSON 516px
Example: http://localhost:3650/api/maps/streets/tiles.json |
tilejson256 | string | URL for tileJSON 256px
Example: http://localhost:3650/api/maps/streets/256/tiles.json |
wms | string | URL for WMS, available only with valid license, must be enabled in settings
Example: http://localhost:3650/api/maps/streets/wms?service=WMS&request=GetCapabilities |
wmts | string | URL for WMTS
Example: http://localhost:3650/api/maps/streets/WMTSCapabilities.xml |
xyz | string | URL for XYZ 512px
Example: http://localhost:3650/api/maps/streets/{z}/{x}/{y}.jpg |
xyz256 | string | URL for XYZ 256px
Example: http://localhost:3650/api/maps/streets/256/{z}/{x}/{y}.jpg |
example
{
"embeddable": "http://localhost:3650/api/maps/streets",
"ogcTiles": "http://localhost:3650/api/maps/streets/tiles",
"stylejson": "http://localhost:3650/api/maps/streets/style.json",
"tilejson": "http://localhost:3650/api/maps/streets/tiles.json",
"tilejson256": "http://localhost:3650/api/maps/streets/256/tiles.json",
"wms": "http://localhost:3650/api/maps/streets/wms?service=WMS&request=GetCapabilities",
"wmts": "http://localhost:3650/api/maps/streets/WMTSCapabilities.xml",
"xyz": "http://localhost:3650/api/maps/streets/{z}/{x}/{y}.jpg",
"xyz256": "http://localhost:3650/api/maps/streets/256/{z}/{x}/{y}.jpg"
}
MapChange Object
Property | Type | Description |
---|---|---|
format | string | New format
Example: jpeg Allowed values:
jpeg
png
webp |
quality | integer | New quality in percent
Example: 92 |
published | boolean | New state of the map serving
Example: true |
example
{
"format": "jpeg",
"quality": 92,
"published": true
}
MapRemove Object
Property | Type | Description |
---|---|---|
removed | array [string] | The names of removed maps
|
example
{"removed": ["streets"]}
MapItemDetailUrls Object
Accessible URLs for the map
Property | Type | Description |
---|---|---|
embeddable | string | URL for the embeddable viewer
Example: http://localhost:3650/api/maps/streets |
ogcTiles | string | URL for OGC API - Tiles
Example: http://localhost:3650/api/maps/streets/tiles |
stylejson | string | URL for styleJSON
Example: http://localhost:3650/api/maps/streets/style.json |
tilejson | string | URL for tileJSON 516px
Example: http://localhost:3650/api/maps/streets/tiles.json |
tilejson256 | string | URL for tileJSON 256px
Example: http://localhost:3650/api/maps/streets/256/tiles.json |
wms | string | URL for WMS, available only with valid license, must be enabled in settings
Example: http://localhost:3650/api/maps/streets/wms?service=WMS&request=GetCapabilities |
wmts | string | URL for WMTS
Example: http://localhost:3650/api/maps/streets/WMTSCapabilities.xml |
xyz | string | URL for XYZ 512px
Example: http://localhost:3650/api/maps/streets/{z}/{x}/{y}.jpg |
xyz256 | string | URL for XYZ 256px
Example: http://localhost:3650/api/maps/streets/256/{z}/{x}/{y}.jpg |
example
{
"embeddable": "http://localhost:3650/api/maps/streets",
"ogcTiles": "http://localhost:3650/api/maps/streets/tiles",
"stylejson": "http://localhost:3650/api/maps/streets/style.json",
"tilejson": "http://localhost:3650/api/maps/streets/tiles.json",
"tilejson256": "http://localhost:3650/api/maps/streets/256/tiles.json",
"wms": "http://localhost:3650/api/maps/streets/wms?service=WMS&request=GetCapabilities",
"wmts": "http://localhost:3650/api/maps/streets/WMTSCapabilities.xml",
"xyz": "http://localhost:3650/api/maps/streets/{z}/{x}/{y}.jpg",
"xyz256": "http://localhost:3650/api/maps/streets/256/{z}/{x}/{y}.jpg"
}
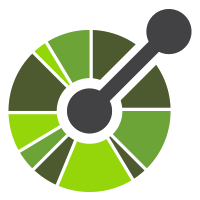
Using the OpenAPI Specification?
Get the openapi.yaml
Server API
Authentication
Server Admin API
On this page